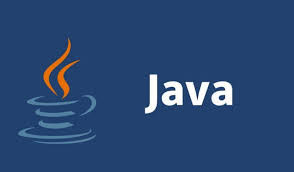
Java is one of the most widely used programming languages globally, celebrated for its flexibility, efficiency, and cross-platform capabilities. This blog provides a thorough guide for beginners to help them master Java. By the end, you'll have a solid grasp of Java's core concepts and be ready to start developing your own projects.
Table of Contents
Introduction to Java
Setting Up Your Development Environment
Basic Syntax and Structure
Object-Oriented Programming (OOP) in Java
Core Java Concepts
Exception Handling
Basic I/O Operations
Collections Framework
Conclusion
Introduction to Java
Java, developed by Sun Microsystems (now owned by Oracle) in the mid-1990s, is a high-level, object-oriented programming language. It was designed to be platform-independent, allowing code to run on any device equipped with the Java Virtual Machine (JVM).
Key Features:
Write Once, Run Anywhere: Java code is compiled into bytecode, which can be executed on any device with a JVM.
Object-Oriented: Java adheres to the object-oriented programming paradigm, encouraging modular and reusable code.
Robust and Secure: Java provides robust security features, exception handling, and memory management.
Multi-threaded: Java supports multithreading, enabling concurrent execution of multiple threads to optimize CPU utilization.
Setting Up Your Development Environment
To begin programming in Java, you need to set up your development environment. Here's how:
Step 1: Install JDK
The Java Development Kit (JDK) includes the JVM, compiler, and other essential tools. Get the most recent version from the Oracle website and install it.
Step 2: Set Up an IDE
An Integrated Development Environment (IDE) simplifies coding by providing features like code completion and debugging. Popular IDEs for Java include:
Eclipse
IntelliJ IDEA
NetBeans
Step 3: Verify Installation
Open your terminal (or command prompt) and use specific commands to check your Java installation to ensure it's correctly set up.
Basic Syntax and Structure
Understanding Java's basic syntax is essential for writing your first program. A typical Java program consists of a class declaration, a main method, and statements that perform actions.
The class serves as a blueprint for objects, while the main method acts as the entry point of the program. Within the main method, statements can include various operations such as printing messages to the console.
Object-Oriented Programming (OOP) in Java
Java is fundamentally object-oriented, meaning it uses objects to represent real-world entities. The following four concepts comprise OOP:
Encapsulation
Encapsulation involves bundling data (variables) and methods (functions) that operate on the data into a single unit, or class. This approach restricts direct access to some components, maintaining data integrity.
Inheritance
By allowing a new class to inherit methods and properties from an existing class, inheritance encourages code reuse and creates a logical hierarchy.
Polymorphism
Polymorphism enables objects to be treated as instances of their parent class rather than their actual class, providing flexibility and allowing for multiple implementations of an interface.
Abstraction
Abstraction involves hiding complex implementation details and exposing only the necessary features, simplifying interactions through clear interfaces and abstract classes.
Core Java Concepts
Variables and Data Types
Java includes various data types, such as integers, floating-point numbers, characters, and boolean values. Variables store data values, and each variable must be declared with a specific data type.
Control Flow Statements
Java supports several control flow statements like conditional statements (if, else, switch) and loop statements (while, for, do-while). These statements control the flow of execution based on conditions or iterations.
Exception Handling
Exception handling in Java is managed through blocks of code designed to handle runtime errors. The try block contains code that might throw an exception, the catch block handles the exception if it occurs, and the finally block executes code regardless of whether an exception was thrown.
This mechanism is crucial for maintaining the robustness of applications by allowing them to handle errors gracefully without crashing.
Basic I/O Operations
Java provides a set of classes and methods for performing input and output operations, such as reading from and writing to the console, files, and other data streams.
For example, the Scanner class is used to read user input from the console, while other classes in the java.io package handle file operations.
Collections Framework
The Java Collections Framework offers a set of classes and interfaces to store and manipulate groups of data as single units. It includes various data structures such as lists, sets, and maps.
Commonly Used Collections:
ArrayList: A resizable array implementation.
HashSet: A set implementation that prevents duplicate elements.
HashMap: A map implementation that associates keys with values.
The framework provides powerful methods for adding, removing, and retrieving elements, making it easier to manage large data collections.
Conclusion
Java is a powerful and versatile programming language suitable for a wide range of applications, from web development to mobile apps and enterprise solutions. This guide provides a foundational understanding of Java's key concepts and syntax. As you continue to learn and practice, you'll be able to build more complex and sophisticated programs. For those looking to accelerate their learning, enrolling in a Java Training Course in Navi Mumbai, Mumbai, Thane, Noida, Delhi and other cities of India can provide structured guidance and hands-on experience.
Comments